Robotic Python Automation – Python to Create Abstract Art Automatically
Looking to spruce up your home office? Here is a quick fun project you can do in Python to Create Abstract Art Automatically to print out for your home! This tutorial uses the basics of working with images, files, math and basic loops to create images you can color in however you like to match the style of your home.
Check out my free Udemy Classes here to learn Python for free! And be sure to check out my books for additional free educational material. Other materials can be found here.
- Create a new Jupyter Notebook in the following location “C:\Users\yourusername\Documents\Python\Training\”
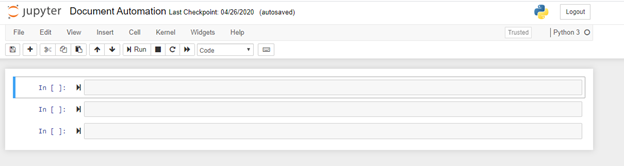
- Create a new folder on your C Drive “C:\Users\yourusername\Documents\Python\Training\art” and create a empty file called “template.png” with a transparent background.
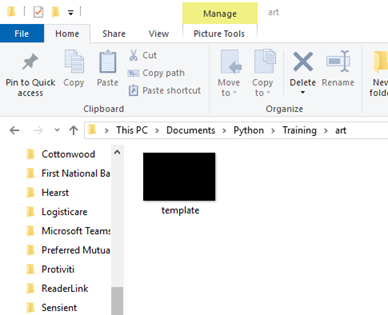
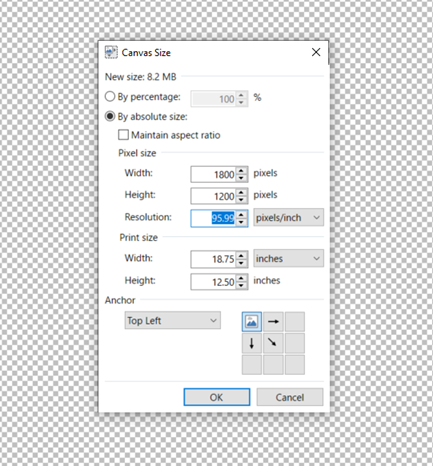
- Install pillow so we can work with images in Python
pip install pillow
- We than use numpy and matplot lib to generate a random shape with the following code. Change some of the constants below to experiment with generating different shapes. The loop (i) can also be changes to generate more or less shapes. This will create 25 shape in Python which you can see in Juptyer and also save them to the art folder.
from matplotlib.path import Path
import matplotlib.patches as patches
import numpy as np
from matplotlib import pyplot as plt
i=1
while i<20:
# UPDATE THESE VARIABLES TO CHANGE THE TYPES OF SHAPES
n = 4 # Number of possibly sharp edges
r = 0.7 # magnitude of the perturbation from the unit circle,
# should be between 0 and 1
N = n*3+1 # number of points in the Path
# There is the initial point and 3 points per cubic bezier curve. Thus, the curve will only pass though n points, which will be the sharp edges, the other 2 modify the shape of the bezier curve
angles = np.linspace(0,2*np.pi,N)
codes = np.full(N,Path.CURVE4)
codes[0] = Path.MOVETO
verts = np.stack((np.cos(angles),np.sin(angles))).T*(2*r*np.random.random(N)+1-r)[:,None]
verts[-1,:] = verts[0,:] # Using this instad of Path.CLOSEPOLY avoids an innecessary straight line
path = Path(verts, codes)
fig = plt.figure()
ax = fig.add_subplot(111)
patch = patches.PathPatch(path, facecolor='none', lw=0.5)
ax.add_patch(patch)
ax.set_xlim(np.min(verts)*1.1, np.max(verts)*1.1)
ax.set_ylim(np.min(verts)*1.1, np.max(verts)*1.1)
ax.axis('off') # removes the axis to leave only the shape
plt.savefig('art/foo'+str(i)+'.png', transparent=True, dpi=300)
i=i+1
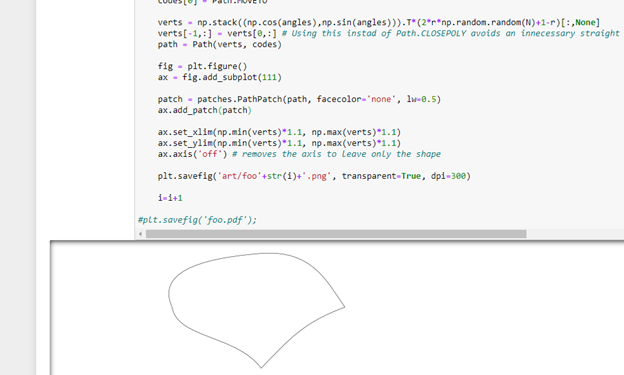
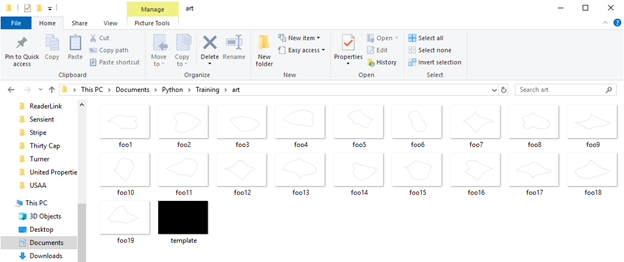
- In our last step all we need to do now is loop through each of the files we created and pasting them into the template image. We use the random function to paste them into random spots on the new image and then save the file as “workofart.png”. This create a cool new pattern on a transparent background!
from PIL import Image, ImageDraw
import numpy as np
import os
import random
i=1
#os.remove("art/workofart.png")
background = Image.open("art/template.png")
while i<20:
foreground = Image.open("art/foo"+str(i)+".png")
background.paste(foreground, (random.randint(-1000,1000), random.randint(-1000,1000)), foreground)
background.save("art/workofart.png")
i=i+1
background.close()
foreground.close()
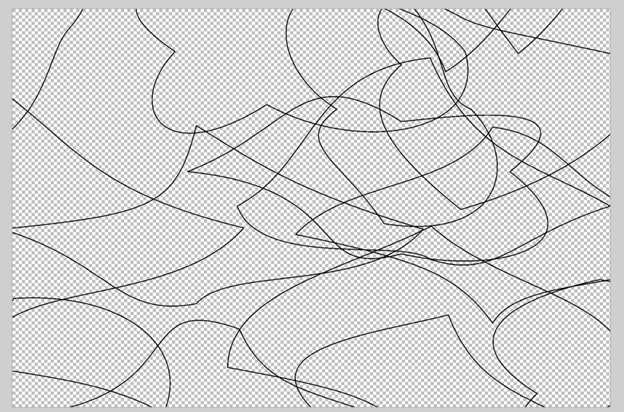
The last step is have some fun. Use your favorite paint colors. Once completed, take over to your local print shop and put in a frame above your desk! Take a look at the artwork I created below.
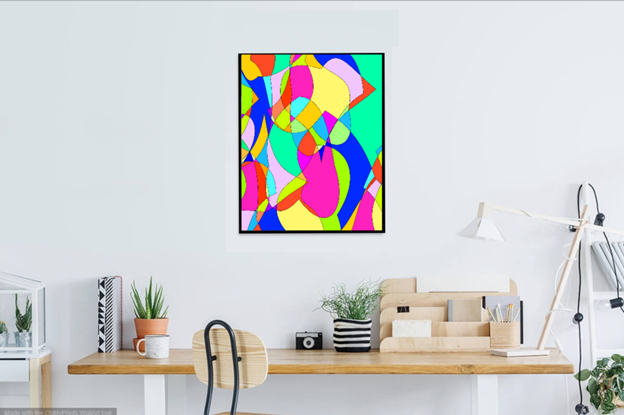
Check out my free Udemy Classes here to learn Python for free! And be sure to check out my books for additional free educational material. Other materials can be found here.
Robotic Python Automation – Python to Create Abstract Art Automatically