Sometimes you do not want to spend money to do things are are really easy. Tools like Hootsuite or Buffer can run you 100’s a month for something you can roll out yourself for free and a hour or so worth of work. Let’s learn how to Automate your Twitter with API and Python
Setting Up Twitter
First we will want to create a Twitter Account and Handle and register for a developer account. This may take a few days for you to get approved.
- Create a Twitter Account
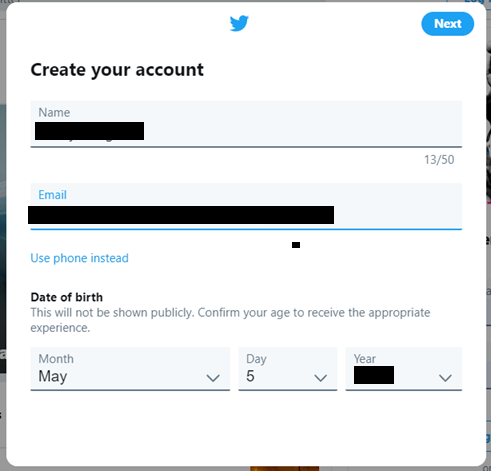
- After a few more steps you will have a working Twitter Account

- Apply for a developer tool. For this we are going to build a hobbyist bot.

- If you have not verified a phone number, it will make you do that now.
- The next few screens will have you fill out why you want to use the portal and review and sign a developers agreement.

- You will than need to wait for your account to be confirmed
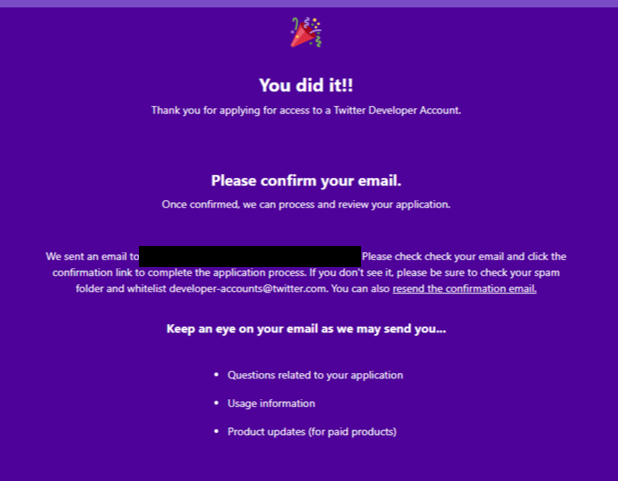
Creating an Twitter App
- Once you app is Confirmed, you can create an app to get an API key
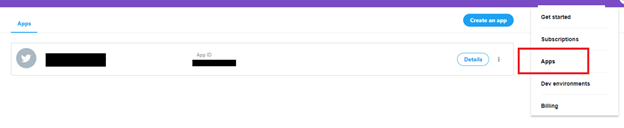
- Obtain your consumer keys and your access tokens, once the app is created
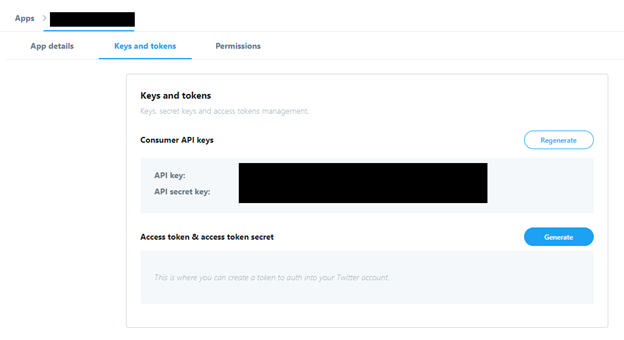
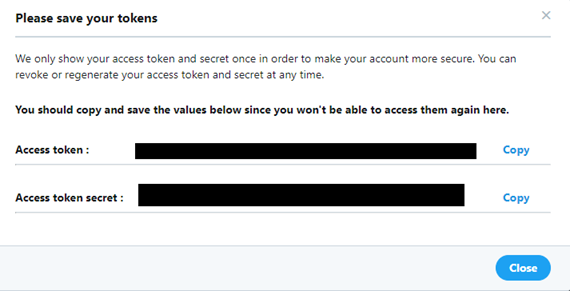
Create a CSV for Data
- Create a CVS File called “post.csv” with your post message, hashtags and urls. If this is not in the same folder as your Python script this will need to be updated in the next steps

Creating a Python Application
- Create a new python application and run the command “pip install tweepy” to install the library
pip install tweepy
- Import your excel file into a dataframe and use the sample command to select a random row. Place each of these values in their own variables.
import pandas as pd
df = pd.read_csv('post.csv')
#print (df)
df2 = df.sample()
title = df2.iloc[0]['Title']
hashtags = df2.iloc[0]['hashtags']
url = df2.iloc[0]['url']
print (title)
print (hashtags)
print (url)

- The following code can now be use to auto post your first twitter message once you replace your keys!
import tweepy
def main():
twitter_auth_keys = {
"consumer_key" : "REPLACE_THIS_WITH_YOUR_CONSUMER_KEY",
"consumer_secret" : "REPLACE_THIS_WITH_YOUR_CONSUMER_SECRET",
"access_token" : "REPLACE_THIS_WITH_YOUR_ACCESS_TOKEN",
"access_token_secret" : "REPLACE_THIS_WITH_YOUR_ACCESS_TOKEN_SECRET"
}
auth = tweepy.OAuthHandler(
twitter_auth_keys['consumer_key'],
twitter_auth_keys['consumer_secret']
)
auth.set_access_token(
twitter_auth_keys['access_token'],
twitter_auth_keys['access_token_secret']
)
api = tweepy.API(auth)
tweet = title + ' ' + hashtags + ' ' + url
status = api.update_status(status=tweet)
if __name__ == "__main__":
main()
Interested in learning more? Check out our blog post on an Introduction to Python Class.
Automate your Twitter with API and Python – Robotic Python Automation